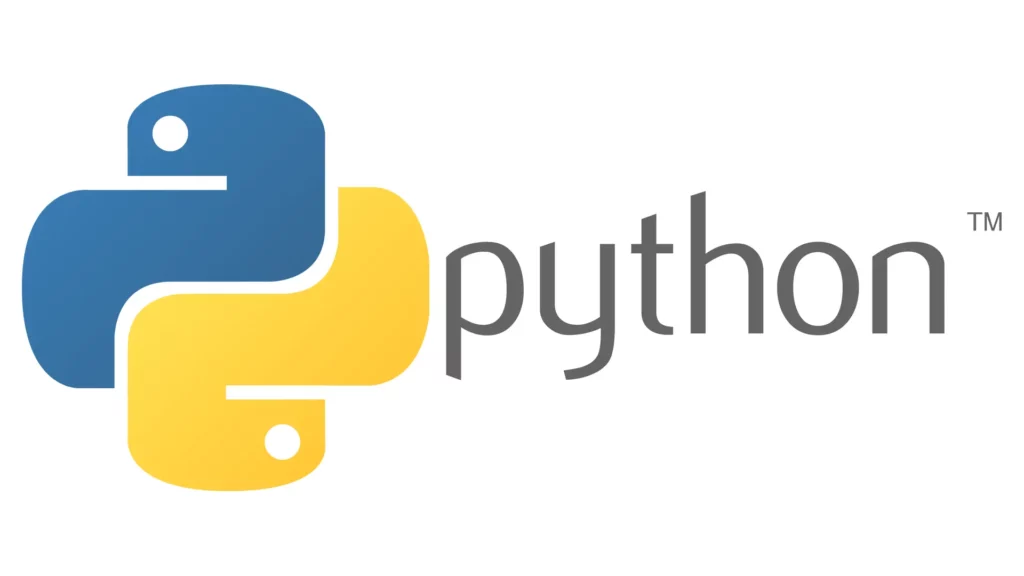
Python has several libraries and wrappers that make it relatively easy to work with OpenGL. Here’s an overview of how to get started with Python and OpenGL:
- Install Dependencies:
- You’ll need Python installed on your system. You can download it from the official Python website.
- Next, you’ll need an OpenGL library. PyOpenGL is a popular choice. You can install it using
pip
:
pip install PyOpenGL
Additionally, you can use a library like GLFW to handle window creation and user input. Install it using pip
:
pip install glfw
Set Up an OpenGL Context:
- You’ll need to create an OpenGL context and a window for rendering. This can be done using GLFW or another windowing library.
import glfw
from OpenGL.GL import *
def main():
# Initialize GLFW
if not glfw.init():
return
# Create a windowed mode window and its OpenGL context
window = glfw.create_window(800, 600, "OpenGL Window", None, None)
if not window:
glfw.terminate()
return
# Make the window's context current
glfw.make_context_current(window)
while not glfw.window_should_close(window):
glClear(GL_COLOR_BUFFER_BIT)
# Add OpenGL rendering code here
glfw.swap_buffers(window)
glfw.poll_events()
glfw.terminate()
if __name__ == "__main__":
main()
- OpenGL Rendering:
- Inside the rendering loop, you can use OpenGL commands to draw 2D or 3D objects. You’ll use the functions provided by PyOpenGL.
- Shaders:
- To render complex scenes, you’ll often need to write shaders in OpenGL. You can use PyOpenGL’s shader support to load and use shaders in Python.
- 3D Models:
- To render 3D models, you may need to use a 3D modeling library like Pygame or Pyglet for loading models, textures, and handling user input.
- Interactivity:
- Handle user input with libraries like Pygame, Pyglet, or the event handling capabilities of the windowing library you’re using.
- Libraries:
- There are Python libraries built on top of OpenGL that simplify 3D graphics programming. One such library is Panda3D, which provides a high-level game engine and 3D graphics framework.
- OpenGL Extensions:
- For advanced OpenGL functionality, you can use PyOpenGL’s extensions to access OpenGL features not covered by the core API.
- Resources and Tutorials:
- There are many tutorials, books, and online resources for learning Python and OpenGL. You can find tutorials on specific topics, like rendering 3D models or creating shaders, to expand your knowledge.
Computer graphics concepts and OpenGL programming is essential for building advanced graphics applications in Python.