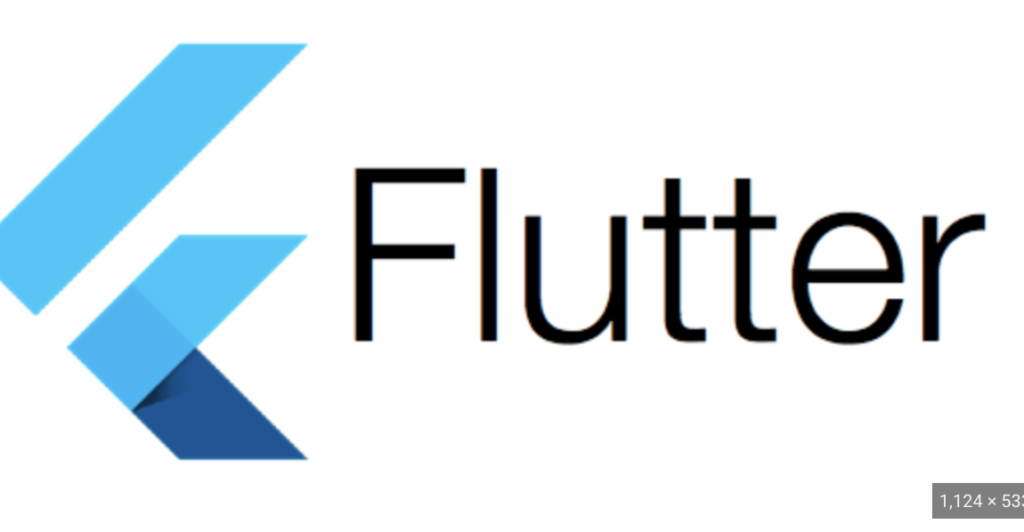
To display a stock chart in a Flutter app, you can use various charting libraries available in Flutter, such as syncfusion_flutter_charts
, flutter_charts
, or fl_chart
. Here’s a basic example using syncfusion_flutter_charts
:
First, add the syncfusion_flutter_charts
dependency to your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
syncfusion_flutter_charts: ^
Once you've added the dependency, you can use the `SfCartesianChart` widget from the `syncfusion_flutter_charts` package to display a stock chart. Here's a simple example:
dart
import ‘package:flutter/material.dart’;
import ‘package:syncfusion_flutter_charts/charts.dart’;
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text(‘Stock Chart Example’),
),
body: Center(
child: Container(
height: 300,
child: SfCartesianChart(
primaryXAxis: DateTimeAxis(),
series: [
CandleSeries(
dataSource: getData(),
xValueMapper: (CandleData data, ) => data.date, lowValueMapper: (CandleData data, ) => data.low,
highValueMapper: (CandleData data, ) => data.high, openValueMapper: (CandleData data, ) => data.open,
closeValueMapper: (CandleData data, _) => data.close,
),
],
),
),
),
),
);
}
List getData() {
return [
CandleData(DateTime(2022, 1, 1), 100, 150, 120, 130),
CandleData(DateTime(2022, 1, 2), 110, 160, 130, 140),
CandleData(DateTime(2022, 1, 3), 120, 170, 140, 150),
// Add more data points as needed
];
}
}
class CandleData {
final DateTime date;
final double low;
final double high;
final double open;
final double close;
CandleData(this.date, this.low, this.high, this.open, this.close);
}
“`
This example displays a simple candlestick chart using the SfCartesianChart
widget from syncfusion_flutter_charts
. Adjust the data and chart configuration as needed for your application.