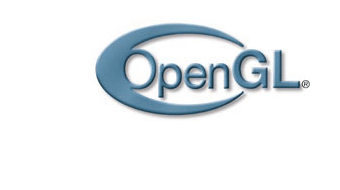
OpenGL is a graphics library primarily used for rendering graphics and is not designed for database operations. If you need to work with databases in combination with OpenGL in an application, you should consider using a database library, such as SQLite or PostgreSQL, alongside OpenGL for rendering.
Here’s a general outline of how you can integrate a database with OpenGL:
- Choose a Database: Select a database system that fits your application’s requirements. Common choices include SQLite for embedded databases, PostgreSQL for more advanced database needs, or other databases like MySQL or MongoDB.
- Database Operations: Write code to perform database operations, such as connecting to the database, executing queries, and retrieving data. You can use database libraries specific to your chosen database system, like SQLite.swift for SQLite or libpq for PostgreSQL.
- Data Management: Retrieve data from the database and store it in a data structure that OpenGL can use for rendering. You might want to create data structures or classes to organize the data effectively.
- OpenGL Integration: Use the data retrieved from the database to render graphics with OpenGL. You can create textures, models, or other OpenGL resources based on the data.
- Display Data: Render the graphics based on the data using OpenGL. You can update the display as needed, for example, by refreshing it when the database data changes.
Here’s a very simplified example that demonstrates how to load textures from a file and display them using OpenGL. In practice, you would retrieve the texture data from the database instead of loading it from a file.
#include <GL/glew.h>
#include <GLFW/glfw3.h>
#include <SOIL/SOIL.h>
#include <iostream>
int main() {
if (!glfwInit()) {
std::cerr << "Failed to initialize GLFW" << std::endl;
return -1;
}
GLFWwindow* window = glfwCreateWindow(800, 600, "OpenGL Database Example", NULL, NULL);
if (!window) {
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
if (glewInit() != GLEW_OK) {
std::cerr << "Failed to initialize GLEW" << std::endl;
return -1;
}
GLuint textureID;
glGenTextures(1, &textureID);
glBindTexture(GL_TEXTURE_2D, textureID);
int width, height;
unsigned char* image = SOIL_load_image("texture.png", &width, &height, 0, SOIL_LOAD_RGB);
if (image) {
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, width, height, 0, GL_RGB, GL_UNSIGNED_BYTE, image);
SOIL_free_image_data(image);
}
while (!glfwWindowShouldClose(window)) {
glClear(GL_COLOR_BUFFER_BIT);
// Render using the loaded texture
glfwSwapBuffers(window);
glfwPollEvents();
}
glfwTerminate();
return 0;
}
In this example, the SOIL
library is used to load an image texture, but you can adapt the concept to load data from a database and use it for rendering. You would replace the file-based texture loading with database retrieval code.