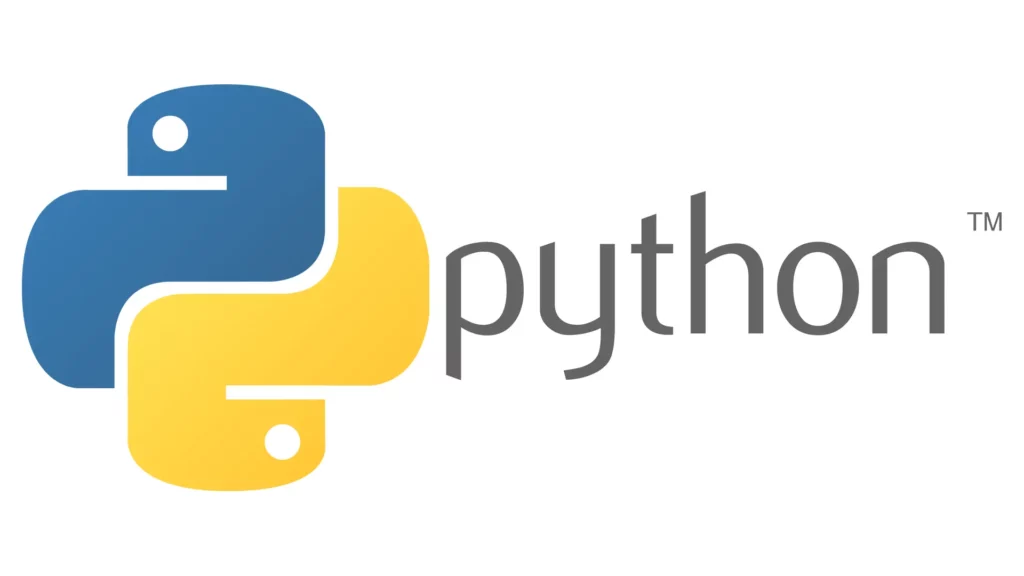
Pyglet is a Python library for creating games and multimedia applications, including 2D and 3D graphics. Here’s a simple example of a Pyglet program that creates a window and displays a rotating 3D cube:
import pyglet
from pyglet.gl import *
# Define the vertices and edges of the cube
vertices = [
-0.5, -0.5, -0.5,
0.5, -0.5, -0.5,
0.5, 0.5, -0.5,
-0.5, 0.5, -0.5,
-0.5, -0.5, 0.5,
0.5, -0.5, 0.5,
0.5, 0.5, 0.5,
-0.5, 0.5, 0.5
]
edges = (
0, 1,
1, 2,
2, 3,
3, 0,
4, 5,
5, 6,
6, 7,
7, 4,
0, 4,
1, 5,
2, 6,
3, 7
)
# Create a Pyglet window
window = pyglet.window.Window()
@window.event
def on_draw():
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
glLoadIdentity()
glTranslatef(0, 0, -3)
glRotatef(30, 1, 0, 0)
glRotatef(30, 0, 1, 0)
pyglet.graphics.draw(len(edges), GL_LINES, ('v3i', vertices), ('i', edges))
pyglet.app.run()
This program creates a Pyglet window and uses OpenGL functions to draw a 3D cube. When you run this program, you should see a rotating cube in the Pyglet window.
Make sure you have Pyglet installed, and you may need to adjust the cube’s rotation and other parameters to suit your requirements