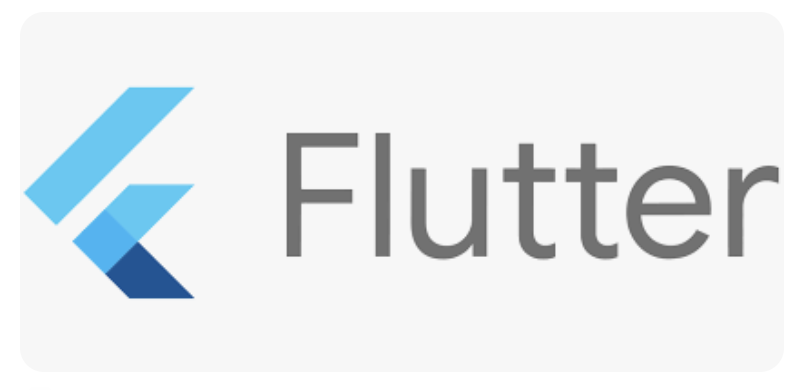
Implementing payment processing in a Flutter application involves integrating a payment gateway or processor to securely handle transactions. Here’s a general guide on how you can achieve this using a popular payment gateway, such as Stripe.
Using Stripe for Payment Processing in Flutter:
1. Create a Stripe Account:
Sign up for a Stripe account if you don’t have one: Stripe Sign Up.
2. Set Up a Flutter Project:
Create a new Flutter project if you haven’t already:
flutter create my_payment_app
3. Add Dependencies:
Open your pubspec.yaml
file and add the Stripe dependencies:
dependencies:
flutter:
sdk: flutter
stripe_payment: ^1.0.9
Run flutter pub get
to fetch the dependencies.
4. Initialize Stripe:
In your Flutter app, initialize Stripe with your publishable key. This should be done in the entry point of your app (e.g., main.dart
):
import 'package:flutter/material.dart';
import 'package:stripe_payment/stripe_payment.dart';
void main() {
StripePayment.setOptions(
StripeOptions(
publishableKey: "YOUR_PUBLISHABLE_KEY",
androidPayMode: 'test', // 'test' or 'live'
),
);
runApp(MyApp());
}
5. Implement Payment Screen:
Create a Flutter screen where users can input their payment details and make a payment. Use the CardField
widget provided by stripe_payment
package:
import 'package:flutter/material.dart';
import 'package:stripe_payment/stripe_payment.dart';
class PaymentScreen extends StatefulWidget {
@override
_PaymentScreenState createState() => _PaymentScreenState();
}
class _PaymentScreenState extends State<PaymentScreen> {
Token _paymentToken;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Payment'),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
CardField(
onCardChanged: (card) {
// Handle card changes
},
onCardSubmitted: (token) {
// Handle submitted card token
setState(() {
_paymentToken = token;
});
},
),
if (_paymentToken != null)
Text('Payment Successful! Token: ${_paymentToken.tokenId}'),
],
),
);
}
}
6. Handle Payment on Server (Optional):
In a real-world scenario, you would send the payment token to your server and handle the payment using the server-side Stripe library. This is to ensure security and compliance.
7. Run Your App:
Run your Flutter app and navigate to the payment screen. Test the payment using the test card details provided by Stripe.
Important Note:
- Ensure you replace
"YOUR_PUBLISHABLE_KEY"
with your actual Stripe publishable key. - Always use test mode during development to avoid actual charges.
- Follow Stripe’s documentation for best practices: Stripe Documentation.
This is a basic example using Stripe, and the exact implementation may vary based on your specific requirements and the payment processor you choose. Always follow security best practices when handling payment information.